'll help analyze this HAR (HTTP Archive) file which contains detailed information about web requests and their performance. Let me break this down into key insights using the analysis tool.View analysisJavascriptExpandCopyconst fileContent = await window.fs.readFile('har.json', { encoding: 'utf8' });
const harData = JSON.parse(fileContent);
// Extract key metrics
const pageData = harData.log.pages[0];
const entries = harData.log.entries;
// Page load timing metrics
const timingMetrics = {
onLoad: pageData.pageTimings.onLoad,
onContentLoad: pageData.pageTimings.onContentLoad,
fullyLoaded: pageData.pageTimings._fullyLoaded
};
// Analyze requests
const requestAnalysis = {
totalRequests: entries.length,
byContentType: {},
totalSize: 0,
failedRequests: 0
};
entries.forEach(entry => {
// Get content type
const contentType = entry.response.content.mimeType.split(';')[0].split('/')[0];
requestAnalysis.byContentType[contentType] = (requestAnalysis.byContentType[contentType] || 0) + 1;
// Calculate size
requestAnalysis.totalSize += entry.response.content.size || 0;
// Count failed requests (4xx and 5xx)
if (entry.response.status >= 400) {
requestAnalysis.failedRequests++;
}
});
// Calculate average response time
const avgResponseTime = entries.reduce((acc, entry) => acc + entry.time, 0) / entries.length;
console.log("Page Load Metrics (ms):", timingMetrics);
console.log("\nRequest Analysis:", requestAnalysis);
console.log("\nAverage Response Time (ms):", avgResponseTime.toFixed(2));
// Analyze failed requests in detail
console.log("\nFailed Requests:");
entries
.filter(entry => entry.response.status >= 400)
.forEach(entry => {
console.log(`${entry.request.url}: ${entry.response.status}`);
});
Result
Page Load Metrics (ms): {"onLoad":2013,"onContentLoad":2013,"fullyLoaded":2651}
Request Analysis: {"totalRequests":32,"byContentType":{"text":29,"font":2,"image":1},"totalSize":768936,"failedRequests":23}
Average Response Time (ms): 333.34
Failed Requests:
https://example.com/hero-image.webp: 404
https://example.com/about-image.webp: 404
https://example.com/feature-icon1.webp: 404
https://example.com/feature-icon3.webp: 404
https://example.com/workshop1.webp: 404
https://example.com/feature-icon2.webp: 404
https://example.com/resource1.webp: 404
https://example.com/formulation-engine.webp: 404
https://example.com/tutorial-icon3.webp: 404
https://example.com/gallery1.webp: 404
https://example.com/resource3.webp: 404
https://example.com/gallery2.webp: 404
https://example.com/workshop2.webp: 404
https://example.com/gallery4.webp: 404
https://example.com/workshop3.webp: 404
https://example.com/gallery5.webp: 404
https://example.com/resource4.webp: 404
https://example.com/gallery3.webp: 404
https://example.com/resource2.webp: 404
https://example.com/tutorial-icon2.webp: 404
https://example.com/footer-logo.webp: 404
https://example.com/tutorial-icon1.webp: 404
https://example.com/gallery6.webp: 404Based on the analysis, here are the key findings:
Page Load Performance:
Initial page load (onLoad): 2,013ms (2.01 seconds)
Content load: 2,013ms
Fully loaded: 2,651ms (2.65 seconds)
Request Statistics:
Total requests: 32
Content type breakdown:
Text (HTML, JavaScript, CSS): 29 requests
Font files: 2 requests
Images: 1 request
Total page size: 768.9 KB
Major Issues:
High number of failed requests: 23 out of 32 requests (71.9%) returned 404 errors
All failed requests are image resources (webp files)
All failed images are trying to load from "example.com" domain, which suggests a configuration issue
Performance Metrics:
Average response time: 333.34ms
Most of the failed requests are image assets that are essential for the page's visual appearance
Recommendations:
Fix the image URLs: All images are pointing to example.com instead of the correct domain
Implement proper error handling for missing images
Consider implementing lazy loading for images to improve initial page load time
Add proper fallback images or placeholder content
Consider implementing a CDN for faster asset delivery
Critical Issues:
The high number of 404 errors will negatively impact:
User experience
Page load performance (wasted requests)
SEO rankings
Server resources
Would you like me to dive deeper into any particular aspect of this analysis or create a visualization of the performance metrics?
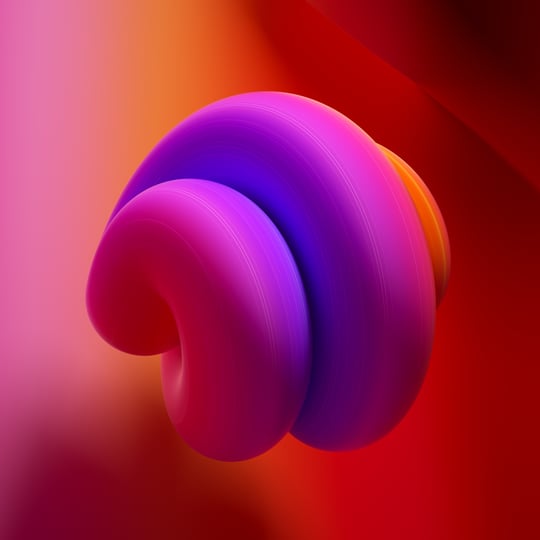